Querying With GraphQL
In addition to the query library, you can also query Irys transactions directly using GraphQL.
Irys vs Arweave GraphQL
This document covers how to query Irys directly, Arweave also has docs on their query schema (opens in a new tab).
GraphQL clients
You can query using an HTTP library like fetch or axios. You can also use specialized clients like Apollo Client (opens in a new tab) or urql (opens in a new tab).
Anatomy of a query
A GraphQL query is made up of:
- Query Arguments: Arguments that specify search parameters, limit the number of results returned, or enable pagination.
- Results Fields: Fields that define the data you want to retrieve.
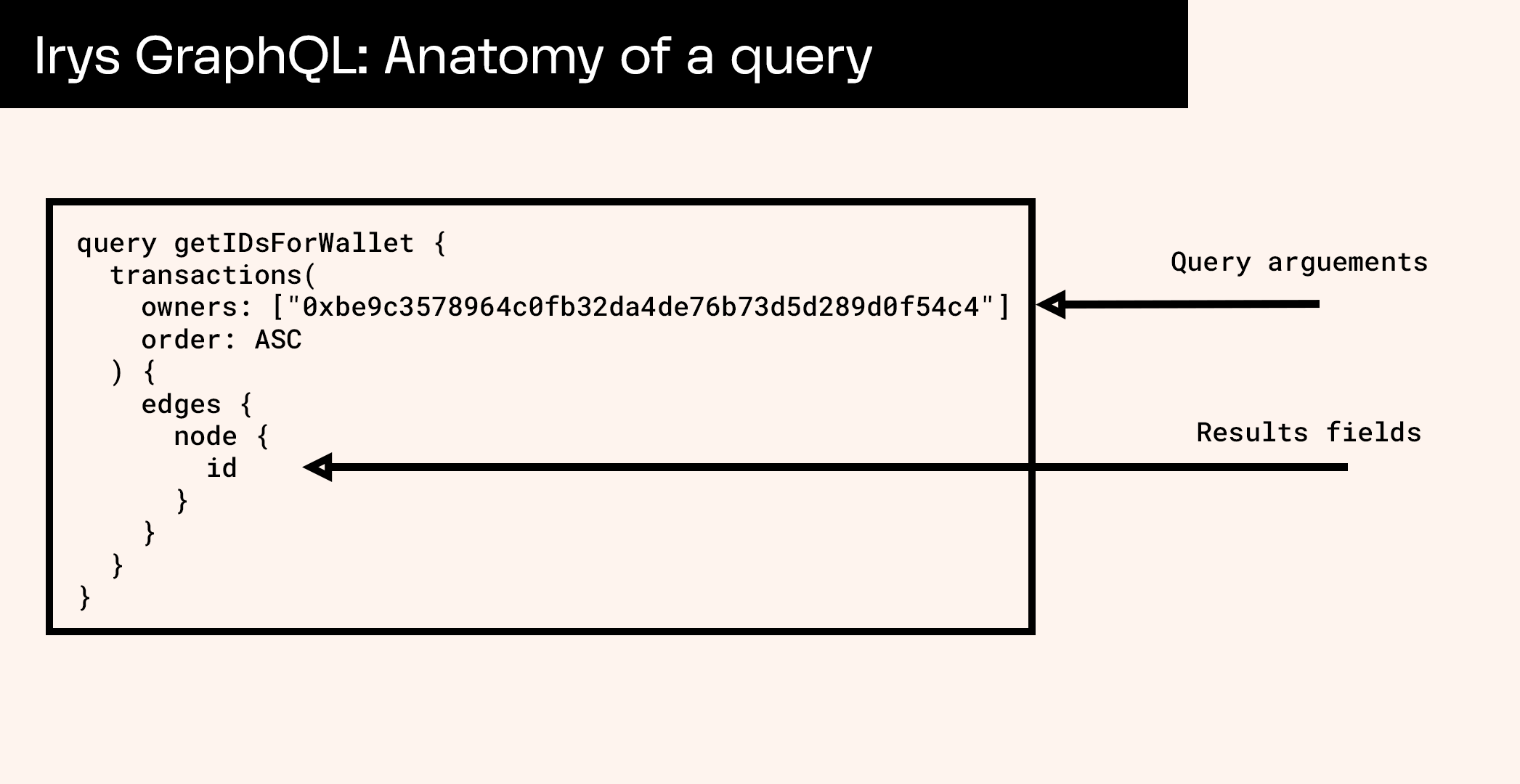
Query arguments
Any of the following query arguments can be used as search parameters:
Field | Description |
---|---|
ids | An array of transaction IDs passed as strings. Values are ORed together, matching results will include transactions that have any of the supplied IDs. |
owner | The address used when posting the transaction. Can be a native address from any of the chains supported by Irys. Note in results fields, this is referred to as address . |
token | The token used to pay for the transaction. |
tags | An array of tag name / value pairs passed as JSON objects. |
Results fields
When building a query, any of the following values be included in your results:
Field | Description |
---|---|
id | The transaction ID. |
address | The address used when posting the transaction. Can be a native address from any of the chains supported by Irys. Note in query arguements, this is referred to as owner . |
token | The token used to pay for the transaction. |
receipt { deadlineHeight signature version } | An optional receipt, only exists if a user requested one at upload.deadlineHeight : The block number by which the transaction must be finalized on Arweave. signature : A signed deep hash of the JSON receipt. |
tags { name value } | An array of tags supplied as name / value pairs. Exists if the user added them at upload. |
timestamp | The timestamp, accurate to the millisecond of when the transaction was posted. This value is the same as the receipt timestamp. |
Mainnet & devnet
We have two GraphQL endpoints, one for mainnet and one for devnet.
Node | Endpoint |
---|---|
Mainnet | https://arweave.mainnet.irys.xyz/graphql (opens in a new tab) |
Devnet | https://arweave.devnet.irys.xyz/graphql (opens in a new tab) |
GraphQL sandbox
Clicking on any of the endpoint URLs above will direct you to the GraphQL Sandbox used for building and testing queries. Press Control+Space at any time to see an interactive popup window of either query arguments or results fields.
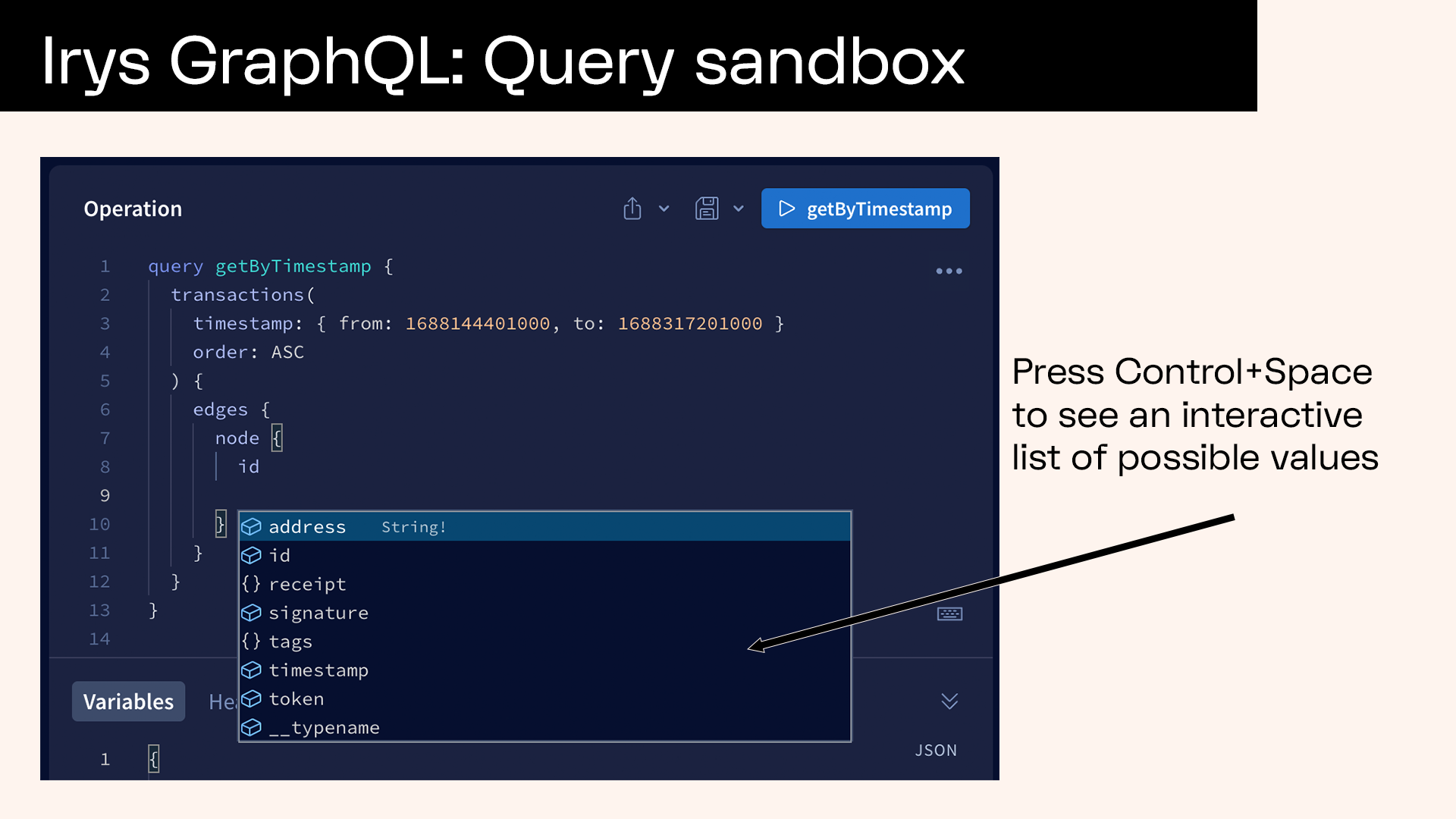
Sample queries
Queries return transaction metadata. To then retrieve data, use the returned transaction ID and download the data from a gateway using a URL formed as follows https://gateway.irys.xyz/[transaction-id]
.
Transaction ids
Search by transaction IDs.
query getByIds {
transactions(ids: ["--52WQHJIJod_rni8pkl1Vxt9MFGoXZAm8SC7ex6C1o", "--52THRWpX_RJzGcNXmtQ2DSP37d1e1VQ4YmvbY5ZXo"]) {
edges {
node {
id
tags {
name
value
}
}
}
}
}
Timestamps
Search by timestamps:
query getByTimestamp {
transactions(timestamp: { from: 1688144401000, to: 1688317201000 }) {
edges {
node {
id
}
}
}
}
Irys timestamps are accurate to the millisecond, so you need to provide a timestamp in millisecond format. You can convert from human-readable time to UNIX timestamp using websites like Epoch101 (opens in a new tab), be sure to convert in millisecond format, not second.
Owners
Search for transactions matching the wallet address used when posting the transaction:
query getByOwner {
transactions(owners: ["0xBcb812C6e26F4F0F78Bd7B6222461FF24F2942AE", "0xaC568a981B1370B2e1bAA8cE30BD5AC9E28C572D"]) {
edges {
node {
id
address
}
}
}
}
Tags
Search for transactions matching tag name / value pairs:
query getAllPNGs {
transactions(tags: [{ name: "Content-Type", values: ["image/png"] }]) {
edges {
node {
id
address
}
}
}
}
Search for transactions matching the tag with name Content-Type and the values of image/png OR image/jpg:
query getTagsWithOR {
transactions(tags: [{ name: "Content-Type", values: ["image/png", "image/jpg"] }]) {
edges {
node {
tags {
name
value
}
}
}
}
}
Search for transactions matching the tag with name Content-Type and the values of image/png AND image/jpg:
query getTagsWithAnd {
transactions(
tags: [{ name: "Content-Type", values: ["image/jpg"] }, { name: "Content-Type", values: ["image/png"] }]
) {
edges {
node {
tags {
name
value
}
}
}
}
}
Limiting results
Limit the number of results returned by including the limit
parameter:
query getAllPNGs {
transactions(limit: 10, tags: [{ name: "Content-Type", values: ["image/png"] }]) {
edges {
node {
id
address
}
}
}
}
Pagination
You can request a maximum of 100 results returned from each query, to obtain additional results use pagination.
When using pagination you:
- Retrieve the
cursor
field, this acts like a bookmark in the search results you can then return to. - Use saved
cursor
value to obtain subsequent search results.
The following query returns 10 transactions tagged image/png
occurring after the cursor with value: LS02d1NsM3R6aUprd3dKUzVjN1FXaWg5aUxsbXh5dVJJbGlydHJtNlpPbw
. To then obtain the next 10 transactions, use the final cursor
value returned from this query as the value of the after
parameter in the following query.
query getPNGs {
transactions(
limit: 10
tags: [{ name: "Content-Type", values: ["image/png"] }]
after: "LS02d1NsM3R6aUprd3dKUzVjN1FXaWg5aUxsbXh5dVJJbGlydHJtNlpPbw"
) {
edges {
node {
id
}
cursor
}
}
}
Sorting
You can sort results by timestamp in either ascending or descending order using the order
field.
query getAllByOwnerAsc {
transactions(owners: ["0xBcb812C6e26F4F0F78Bd7B6222461FF24F2942AE"], order: ASC) {
edges {
node {
id
address
}
}
}
}
query getAllByOwnerDesc {
transactions(owners: ["0xBcb812C6e26F4F0F78Bd7B6222461FF24F2942AE"], order: DESC) {
edges {
node {
id
address
}
}
}
}