Ordering
Irys enables users to instantly sequence data with extreme precision.
This supports applications that require a guarantee on order such as execution layers (rollups), messaging and event processing, among others.
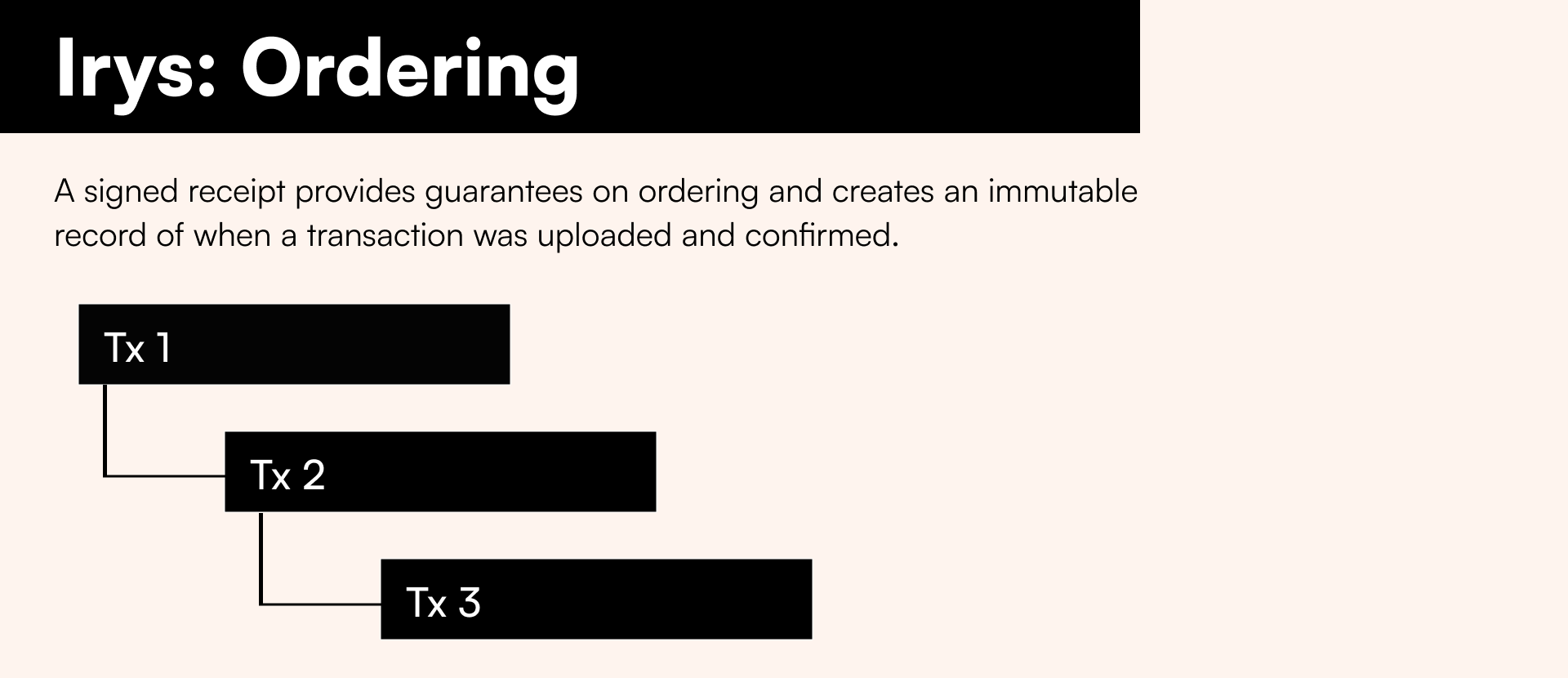
Proof of time
Each transaction comes with a signed receipt that includes a verifiable timestamp, which is accurate to the millisecond. With this, users can always rely on the chronology of their data.
Search seamlessly
Tags categorize transactions. Timestamps order them. Together, tags and timestamps enable users to categorize and order their data to efficiently search for data with single queries.
Getting started
You can upload multiple transactions and sequence them using our SDK and query package in a few lines of code.
Uploading
// Connect and fund
const irys = new Irys({ network, token, key });
const fundTx = await irys.fund(irys.utils.toAtomic(0.05));
// Tag your uploads
const tags = [{ name: "application-id", value: "my-tx-sequence" }];
// Upload 10 transactions
for (let i = 0; i < 10; i++) {
const receipt = await irys.upload("GM " + i, { tags: tags });
console.log(`Transaction #${i} uploaded at ${receipt.timestamp}`);
}
Ordering
const myQuery = new Query();
const results = await myQuery
.search("irys:transactions")
.tags([{ name: "application-id", values: ["my-tx-sequence"] }])
.sort("ASC")
.stream();
// Iterate over the results
for await (const result of stream) {
console.log(result);
}