Irys SDK tutorial
In this tutorial you will learn how to use Irys with NodeJS to permanently store data with strong provenance using a few lines of code.
Prerequisites
To use this tutorial, you will need:
- A crypto wallet with a small amount of Devnet tokens (opens in a new tab)
- A recent version of NodeJS (opens in a new tab)
- A code editor (VSCode (opens in a new tab) or others)
Installing
First, make sure you have Node installed:
node -v
If you get a version number like "v16.14.0" back, you’re good to go. Otherwise, install Node (opens in a new tab).
Next, create a new directory and cd into it.
mkdir irys-basics
cd irys-basics
And then install the SDK using npm:
npm install @irys/sdk
or using yarn:
yarn add @irys/sdk
When you use npm or yarn to install the SDK, it will create a file titled package.json
in your root directory. Open up that file and add "type" : "module"
to the beginning, allowing you to use ES6 module imports.
Finally, run the following in your command prompt to create a new JavaScript file, then fire up your code editor of choice.
touch irys-basics.js
dotenv
Install the free dotenv
package. We will store our private key in a .env
file and access it using the dotenv
package.
npm install dotenv
Now create a file called .env
in your main directory:
touch .env
And edit it to contain the private key of a wallet file that has been funded with free Sepolia tokens (opens in a new tab).
ETH_PRIVATE_KEY=________
Connecting to Irys
Start by connecting to Irys. In this example, we connect to the Irys devnet, which also requires you to pass an RPC URL. When connecting to Irys' mainnet, you don't need to supply an RPC address.
const getIrys = async () => {
const network = "devnet";
// Devnet RPC URLs change often, use a recent one from https://chainlist.org/
const providerUrl = "";
const token = "ethereum";
const irys = new Irys({
network, // "mainnet" || "devnet"
token, // Token used for payment
key: process.env.ETH_PRIVATE_KEY, // Private key
config: { providerUrl }, // Optional provider URL, only required when using Devnet
});
return irys;
console.log(`wallet address = ${irys.address}`);
};
await getIrys();
Paste the above code into the irys-basics.js
file you created and then run it by typing:
node irys-basics.js
from your CLI, if it successfully prints your wallet address, you’re good to go.
Funding a node
To fund a node, pass the amount you want to fund in atomic units. Assuming you setup Irys to use Mumbai Matic, the following will fund 0.5 MATIC:
const fundNode = async () => {
const irys = await getIrys();
try {
const fundTx = await irys.fund(irys.utils.toAtomic(0.5));
console.log(`Successfully funded ${irys.utils.fromAtomic(fundTx.quantity)} ${irys.token}`);
} catch (e) {
console.log("Error uploading data ", e);
}
};
await fundNode();
Paste the above code into irys-basics.js
and run as you did before.
Checking upload price
You can check the cost to upload data by passing a number of bytes to the function irys.getPrice()
. This code checks the cost to upload 1 Megabyte (1048576 bytes).
const checkPrice = async () => {
const irys = await getIrys();
const numBytes = 1048576; // Number of bytes to check
const priceAtomic = await irys.getPrice(numBytes);
// Convert from atomic units to standard units
const priceConverted = irys.utils.fromAtomic(numBytes);
console.log(`Uploading ${numBytes} bytes costs ${priceConverted}`);
};
await checkPrice();
Uploading data
If it can be reduced to 1s and 0s, it can be uploaded to Arweave via Irys using the function irys.upload()
, as with this example that uploads the string "GM world."
const uploadData = async () => {
const irys = await getIrys();
const dataToUpload = "GM world.";
try {
const receipt = await irys.upload(dataToUpload);
console.log(`Data uploaded ==> https://gateway.irys.xyz/${receipt.id}`);
} catch (e) {
console.log("Error uploading data ", e);
}
};
await uploadData();
Uploading a file
You can upload a file by passing its name to the function irys.uploadFile()
. To run this code, find a PNG file on your local drive, rename it to myImage.png
and place it in the same directory as the irys-basics.js
file.
const uploadFile = async () => {
const irys = await getIrys();
// Your file
const fileToUpload = "./myImage.png";
const tags = [{ name: "application-id", value: "MyNFTDrop" }];
try {
const receipt = await irys.uploadFile(fileToUpload, { tags: tags });
console.log(`File uploaded ==> https://gateway.irys.xyz/${receipt.id}`);
} catch (e) {
console.log("Error uploading file ", e);
}
};
await uploadFile();
Uploading a folder
You can also upload an entire folder of images with a single line of code. To run this example, create a directory in the same folder as irys-basics.js
and fill it with image files.
const uploadFolder = async () => {
const irys = await getIrys();
// Upload an entire folder
const folderToUpload = "./my-images/"; // Path to folder
try {
const receipt = await irys.uploadFolder("./" + folderToUpload, {
indexFile: "", // optional index file (file the user will load when accessing the manifest)
batchSize: 50, //number of items to upload at once
keepDeleted: false, // whether to keep now deleted items from previous uploads
}); //returns the manifest ID
console.log(`Files uploaded. Manifest Id ${receipt.id}`);
} catch (e) {
console.log("Error uploading file ", e);
}
};
await uploadFolder();
Files uploaded via irys.uploadFolder(folderToUpload)
can be retrieved in one of two ways.
- Creating a URL with the format
https://gateway.irys.xyz/[manifest-id]/[original-file-name]
. - Creating a URL using the transaction ID of each individual file uploaded with the format
https://gateway.irys.xyz/[transacton-id]
After a successful folder upload, two files are written to your local project directory [folder-name].csv
and [folder-name].json
. The example below highlights a folder called “llama_folder” with a total of 5 files in it. The transaction id for each file can be used to retrieve the uploaded data by forming a URL with the format https://gateway.irys.xyz]/[transaction-id]
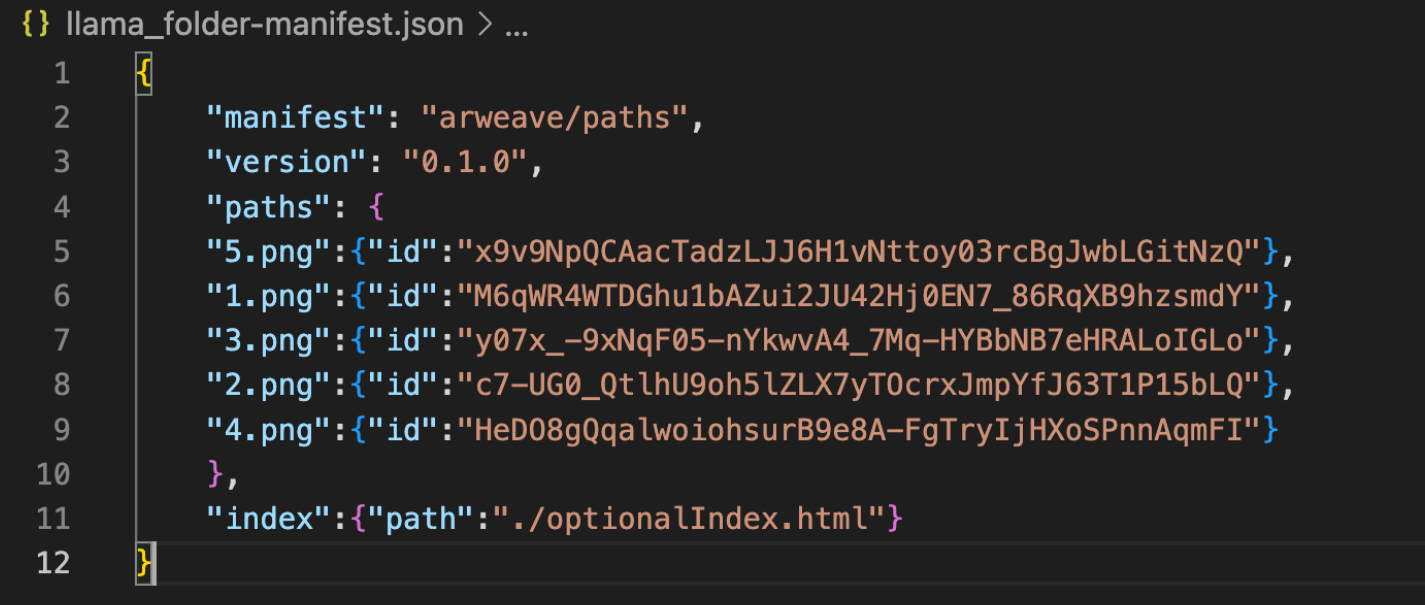
Uploading a static website
You can also use the SDK to uplaod an entire static website to the permaweb.
Getting help
If you get stuck or need help, reach out in our Discord (opens in a new tab) and someone will get back to you quickly.
And, most importantly pleaase let us know how you're using Irys and what you're building. Let us know how we can best support you!